Introduction
Welcome to Summarize Reviews! Making informed purchasing decisions has never been easier. At SummarizeReviews.com, we harness the power of AI to analyze countless product reviews and deliver clear, concise summaries tailored to your needs. Whether you're shopping for gadgets, household essentials, or the latest trends, our platform provides you with quick, actionable insights—saving you time and effort while ensuring confidence in your choices. Say goodbye to review overload and hello to smarter shopping!
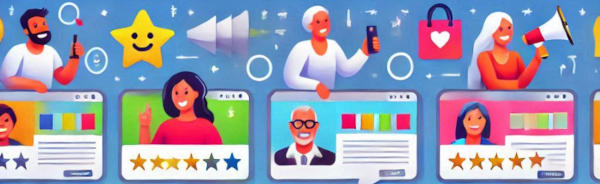
Product Category Search
Top rated data structure and algorithms
Here are some top-rated data structures and algorithms, widely used and accepted in the field of computer science:
Data Structures:
- Arrays: A fundamental data structure used to store a collection of elements of the same data type.
- Linked Lists: A dynamic data structure used to store a collection of elements, where each element is a separate object.
- Stacks: A Last-In-First-Out (LIFO) data structure used to store and retrieve elements.
- Queues: A First-In-First-Out (FIFO) data structure used to store and retrieve elements.
- Trees: A hierarchical data structure used to store elements in a tree-like structure.
- Graphs: A non-linear data structure used to store elements and their relationships.
- Hash Tables: A data structure used to store key-value pairs and provide fast lookup, insertion, and deletion operations.
Algorithms:
- Sorting Algorithms:
- Quicksort: A divide-and-conquer algorithm with an average time complexity of O(n log n).
- Merge Sort: A divide-and-conquer algorithm with a time complexity of O(n log n).
- Heap Sort: A comparison-based sorting algorithm with a time complexity of O(n log n).
- Searching Algorithms:
- Linear Search: A simple searching algorithm with a time complexity of O(n).
- Binary Search: A fast searching algorithm with a time complexity of O(log n).
- Graph Algorithms:
- Dijkstra's Algorithm: A shortest path algorithm with a time complexity of O(|E| + |V|log|V|).
- Bellman-Ford Algorithm: A shortest path algorithm with a time complexity of O(|E| * |V|).
- Floyd-Warshall Algorithm: A shortest path algorithm with a time complexity of O(|V|^3).
- String Algorithms:
- Rabin-Karp Algorithm: A string searching algorithm with a time complexity of O(n + m).
- Knuth-Morris-Pratt Algorithm: A string searching algorithm with a time complexity of O(n + m).
- Dynamic Programming:
- Fibonacci Series: A classic example of dynamic programming with a time complexity of O(n).
- Longest Common Subsequence: A dynamic programming algorithm with a time complexity of O(n * m).
Top-Rated Algorithms:
- Topological Sort: A linear ordering of vertices in a directed acyclic graph (DAG).
- Minimum Spanning Tree: A subset of edges in a graph that connects all vertices with minimum total weight.
- K-Means Clustering: An unsupervised learning algorithm for partitioning data into K clusters.
- Sieve of Eratosthenes: An algorithm for finding all prime numbers up to a given number.
- Fast Fourier Transform: An efficient algorithm for calculating the discrete Fourier transform of a sequence.
Note: The time complexities mentioned above are for the average case, and the actual time complexity may vary depending on the specific implementation and input data.